JavaScript is by far the most fast-paced ecosystem in the world of software development. Things are constantly changing, and the tools that were hot yesterday are on their way out today. That’s doubly true for React libraries. While it can get tiresome, you have to keep up with the changes to keep your skills relevant and productivity high.
So let’s cover the alternatives to the most popular React libraries that are in a lot of ways a better choice.
moment VS date-fns
Let’s start with a heavyweight of the JavaScript world. MomentJS has been around for a long time and is one of the most widely used libraries out there.
MomentJS is a monster with a lot of features included. The downside is that this makes the size of the library pretty big.
The bundle size of MomentJS is 288kB – that’s a lot for some date manipulation. Especially considering that you can’t import individual methods, which means you can’t do any tree-shaking with it.
Another reason you should reconsider using MomentJS is that the development of the library has been stale. Even the creators of MomentJS announced that they stopped shipping new features.
date-fns is another popular date manipulation library. The library has most of the same features yet a much smaller bundle size:
date-fns returns native JavaScript date objects, which makes things easier. You will no longer have to juggle between moment and native date formats. Here’s a sample code of formatting dates with date-fns:
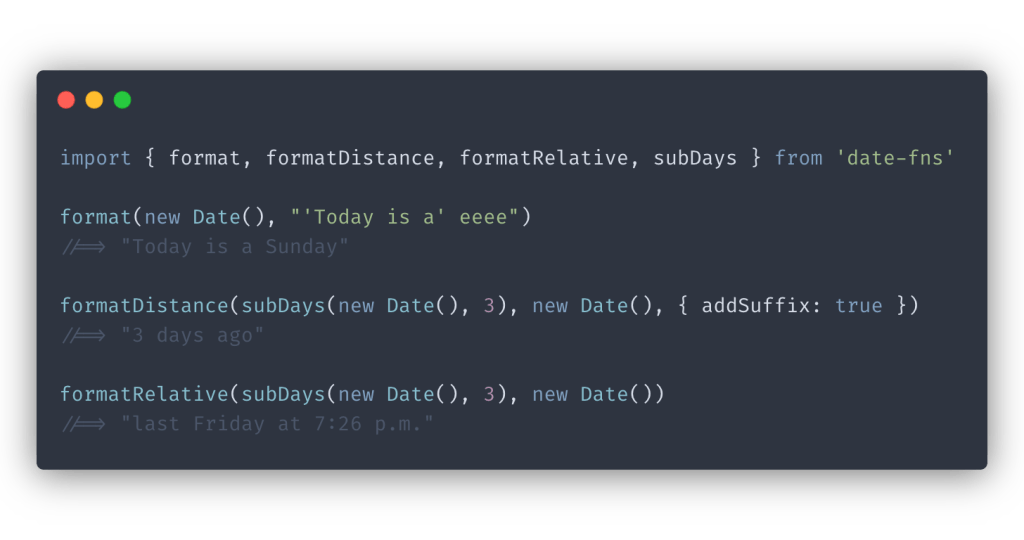
On top of that, the date-fns library is immutable. That means that it returns a new instance of the date rather than mutating the one you give it.
Overall, date-fns is a solid substitution to MomentJS.
Formik VS React Hook Form
Formik is one of the most popular React libraries for handling forms. At the time of writing, it has almost 27K stars on Github. Formik is a very mature library with tons of functionality for handling the main pain points of building a form.
With that said, Formik has one main disadvantage – excessive re-renders. Formik will keep re-rendering the whole form as the user types. If you have heavy UI in your form, Formik will bring your app to its knees by re-rendering the form on each keystroke.
React Hook Form is a newer library for handling forms. As the name suggests, it’s built with hooks. Overall, React Hook Form takes a leaner approach to building forms, and you will end up writing fewer lines of code with it.
But the main advantage of React Hook Form over Formik is that it uses an uncontrolled input approach which saves your app from those redundant re-renders.
Connecting the form is easy and unintrusive: all you need to do is register your form’s inputs using the register
method and wrap the submit event handler with handleSubmit
.
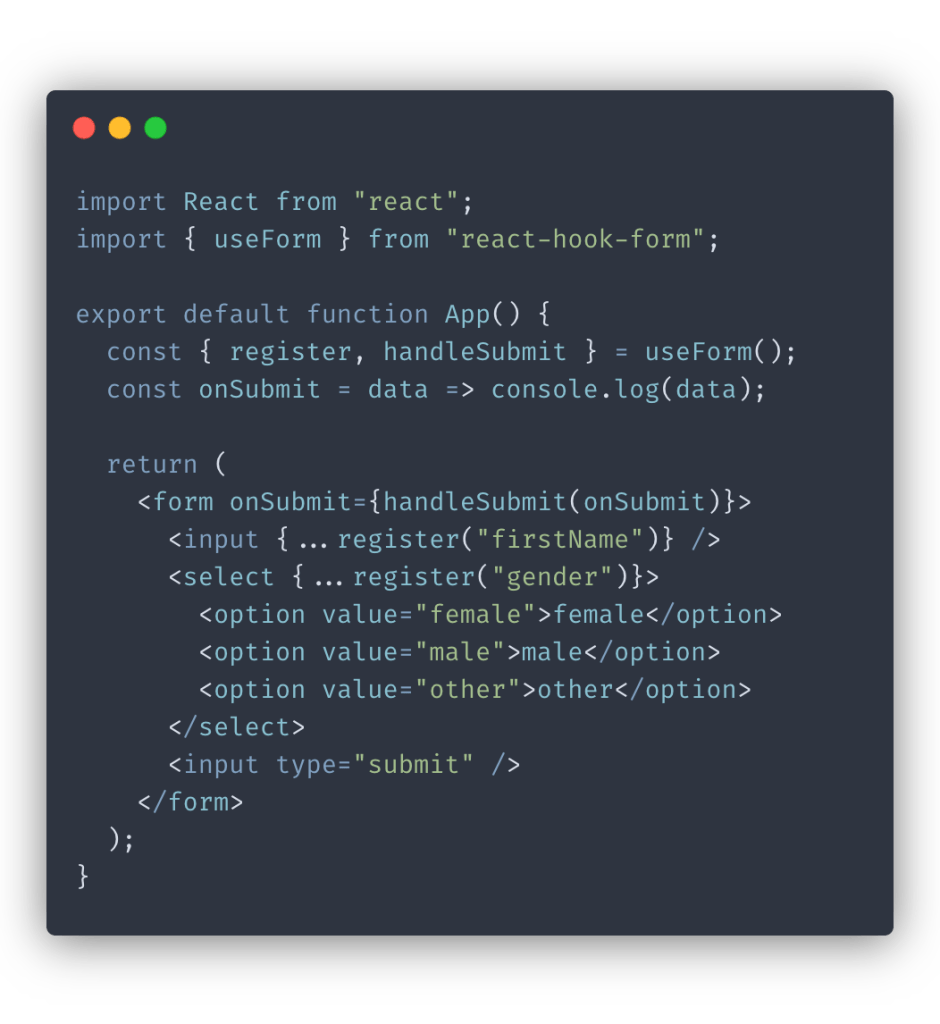
React-router vs HookRouter
React-router is the go-to React library for managing routing in your app. The problem I had with react-router in the past is how bloated it was. But, with the introduction of the hooks, the authors of react-router added hooks to the API. Hooks significantly reduced the amount of boilerplate code you write. Still, there are some interesting alternatives out there.
hookrouter is a newer library entirely based on hooks that takes a lighter approach to app routing. hookrouter has most of the basic features react-router has like programmatic navigation, redirects, and passing route props.
Rather than declaratively defining your routes using Route
and Switch
, you use the useRoutes
hook that consumes your routes
object, evaluates each route one by one, and checks if they match the current URL path.
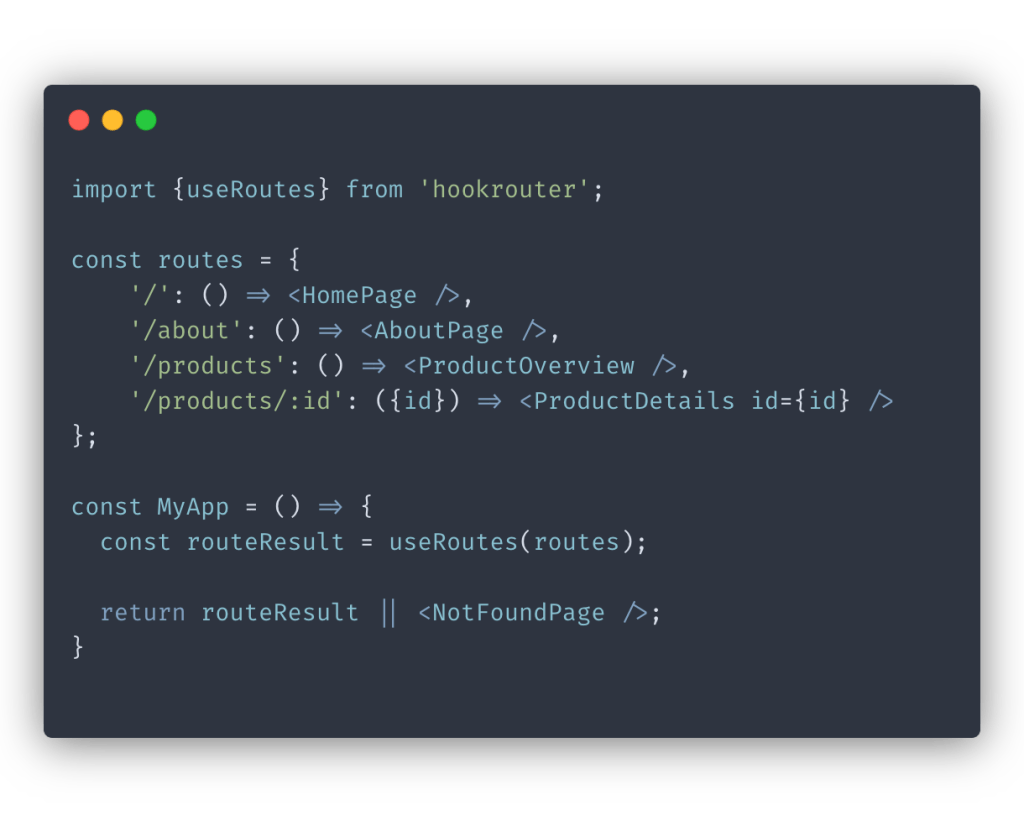
With hookrouter, the nesting of the routes is simpler. If you use the useRoutes
hook inside a child component, it will not match against the full URL path but only against the part of the URL left after removing the parent’s path. That makes it easier to reason about nesting.
So which one is better? In this case, there is no clear winner, and the answer is it depends.
react-router still has more functionality and might be a better choice for larger projects.
You might like hookrouter better if you’re a fan of the hook-based approach. It’s also a better tool for smaller apps where using react-router is overkill.
Lodash VS Native JavaScript
Lodash is a great library that provides a lot of features JavaScript used to lack. However, JavaScript has been continuously improving. With the release of ECMAScript2015, some of the most used functionality of Lodash is now natively supported.
That is not to say that Lodash is entirely obsolete. It still has a lot of very useful APIs that aren’t worth reinventing. Just be aware that there’s a pretty good chance you could omit Lodash from your project and have fewer dependencies as a result.
For example, here’s how you can use native JavaScript to do what the fromPairs function does:
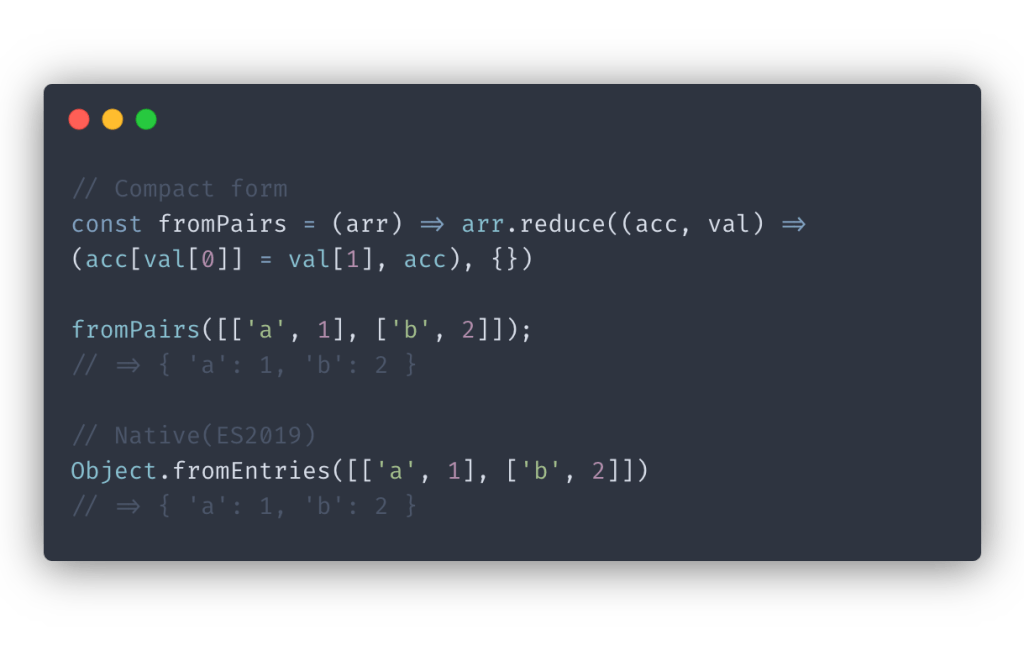
Object.fromEntries
is a new utility method from the ES2019 proposal that does the same thing as fromPairs
. All of the modern browsers support it, except for IE and Opera. If you have to support older browsers, you can easily recreate the functionality using array’s reduce
method.
Here’s a great curated list of examples of using native JavaScript utilities over Lodash.
And that’s it for this post. I hope you found this list of React libraries useful. Keep in mind that this was not a strictly 1:1 comparison. These alternatives are not necessarily better in every situation, so you should still use good judgment and use what suits your project better.
If you’d like to get more web development, React and TypeScript tips consider
following me on Twitter
, where I share things as I learn them.
Happy coding!