Introduction
JavaScript decorators are a relatively new feature in JavaScript that allows adding new behavior to existing functions and objects without modifying their underlying code. You can achieve it by using a special syntax that uses an @ symbol before the function name or object key. Let’s take a quick look at what they are and how they work.
As a side note, decorators aren’t yet a standard feature of JavaScript; they’re still in the ECMA TC39 specification phase . That means we will need to transpile our code with Babel
In general terms, decorators can be thought of as wrappers around other pieces of code that provide additional functionality either on top of it or instead of it. Decorators are not exactly new ideas; in fact, their functionality is similar to that of higher-order functions .
You may apply decorators to class fields , methods, and even the whole class. However, you can’t use decorators on plain JavaScript objects; they only work with classes.
Now let’s see how we can use decorators to decorate properties and methods of our classes.
Decorating class fields
Here is how that might look like using a decorator syntax on a class field:
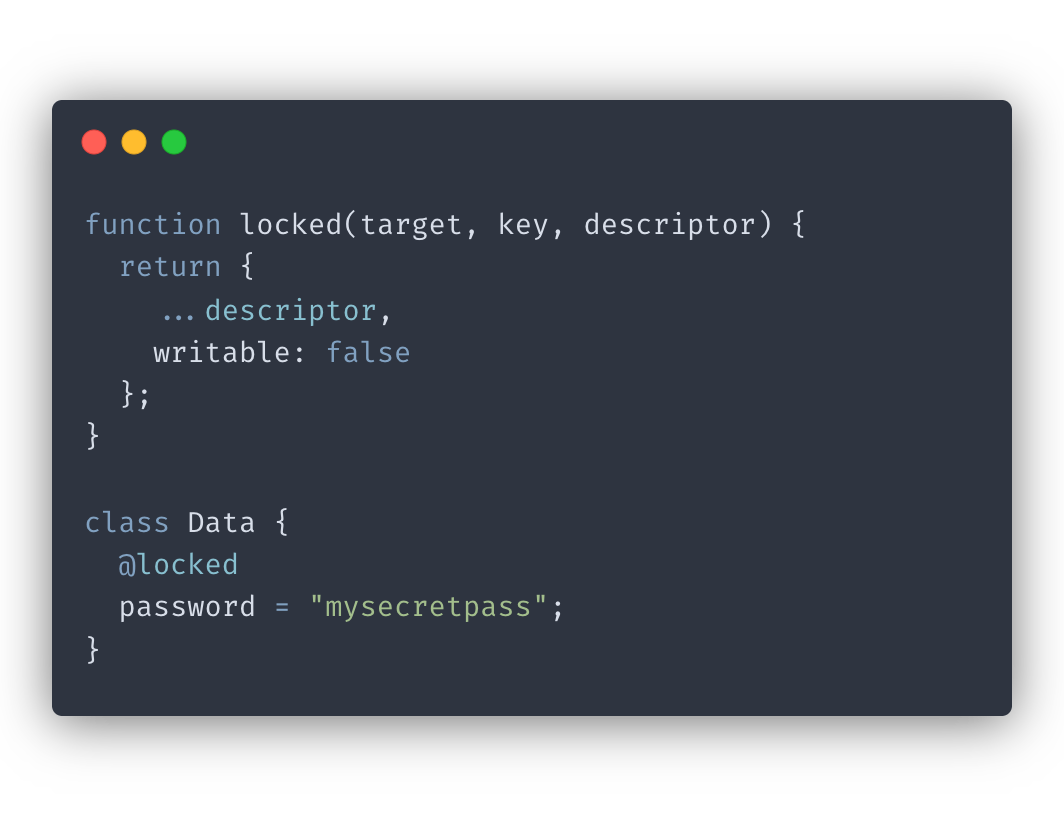
In the code above, we have a simple decorator function called locked
that we apply to the password
class field. It takes in three parameters - target, key, and
descriptor
. The target
is the object or function that is being decorated and the key
refers to the property name on that target. The descriptor contains all of the properties of the target, including those that are being decorated.
Class field decorators work by returning a new descriptor. So we return a new descriptor object with all of the properties and values from the original except the writable
flag set to false
. This prevents changing thepassword
field after it’s been instantiated.
Now if we try changing our password
field:
As we can see, attempting to change the password
field causes an error because now it’s a read-only field.
Decorating class methods
Now, let’s take a look at an example of using decorator on a method instead:
As we can see, decorators for methods work by taking a descriptor and returning an object that contains the original method plus any additional logic.
In this case, we are adding some error handling to our fetchData
method. If anything goes wrong with our request or parsing of the data, it will be caught and logged to the console.
Decorating a class
Now let’s see how we can apply a decorator to the whole class:
We can see that the @saveInCache
decorator is applied to our class, and it will automatically save any new instances of Person
in a cache map.
Conclusion
JavaScript decorators are a powerful feature that can save you time and reduce redundancy in your code. Decorators are still in the proposal stage, so their syntax a subject to change. Keep that in mind before applying adding them to your project.
There are many different ways you can use decorators in your code - either on classes, methods, or properties. Decorators provide an easy way to add additional behavior or metadata in a reusable way without modifying the existing code.
If you’d like to get more web development, React and TypeScript tips consider
following me on Twitter,
where I share things as I learn them.
Happy coding!